Archive for the ‘Uncategorized’ Category
October 13, 2022
We can format the date in groovy without importing the java simple date formats. Simply use like this :
String oldDate = '01/10/2022 13:48'
Date date = Date.parse( 'MM/dd/yyyy HH:mm', oldDate )
String newDate = date.format( 'yyyy-MM-dd' )
println newDate
Posted in Uncategorized | Leave a Comment »
February 16, 2022
If you want to convert decimal values into hours and minutes, and you are stuck with XSLT, use this.
<xsl:stylesheet version="3.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="text"/>
<xsl:variable name="decimal_hours" select="3.14"/>
<xsl:template match="/">
<xsl:value-of select="concat(
format-number(floor($decimal_hours ), '00:'),
format-number(floor($decimal_hours * 60 mod 60), '00:'),
format-number(floor($decimal_hours * 360 mod 360), '00'))"/>
</xsl:template>
</xsl:stylesheet>
Tags:decimal, hours, sap cloud platform integration, stylesheet, time, XSLT, xslt mapping
Posted in Uncategorized | Leave a Comment »
December 3, 2021
In order to upsert into an MDF Object which have attachment, first you have to upsert into the Attachment entity with the base64 encoded File String. This upsert will return the attachment ID. Then retrieve the attachment ID from the response and upsert into the MDF object. The attachment id can be retrieved with XPatch expression like this.
<uri>Attachment(attachmentId=<xsl:copy-of select="substring-after(//atom:entry/atom:content/m:properties/d:key,'Attachment/attachmentId=')"/>)</uri>
After this, Upsert the attachment using this attachment ID wtih payload looking like this:
{
"cust_ParPaySlip_externalCode": "bbbb",
"cust_PayrollPeriod": "/Date(1625316265000)/",
"cust_AttachmentNav": {
"__metadata": {
"uri": "Attachment(attachmentId=16840)"
}
},
"__metadata": {
"uri": "cust_ListPaySlip"
}
}
Tags:attachment, mdf, odata, successfactors, successfactors integration
Posted in Uncategorized | Leave a Comment »
November 17, 2021
To format the datetime in XSLT, first convert it to date time, and use the XSLT function format-dateTime. For example use this to format to the pattern yyyy-MM-dd. Have fun 🙂
<HireDate>
<xsl:value-of select="format-dateTime(xs:dateTime(startDate),'[Y0001]-[M01]-[D01]')"/>
</HireDate>
Posted in Uncategorized | Leave a Comment »
May 17, 2017
Was struggling with building my project using gradle for some time. Whenever i tried to build it, i get an error message “Could not find tools.jar. Please check that C:\Program Files\Java\jre1.8.0_131 contains a valid JDK installation.”. I made it double sure that my standard JVM is pointing to the JDK installation folder, not the JRE folder. Still getting the error, later I found out that gradle is taking the java.home for the build process. For that you can either start the eclipse through command line parameter for java.home.
Or the easy solution i found is, go to the gradle task you want to run, right click, go to gradle configuration, and in the Java Home tab, point to the JDK installation folder. Voila, it will build the project without crying.
Happy Coding guys!
Tags:could not find tools.jar, gradle, java, java.home, jdk, jre, tools.jar
Posted in java, Uncategorized | Leave a Comment »
February 14, 2017
While debugging Java code, Strings in the views “Variables” and “Expressions” show up only till a certain length, after which Eclipse shows “…”. Even after working with eclipse for 10 years it was a new info for me. There is a way to show the complete string.
In the Variables view you can right click on Details pane (the section where the string content is displayed) and select “Max Length…” popup menu. The same length applies to expression inspector popup and few other places. In the max length give 0 and you will see the whole values in the value box.
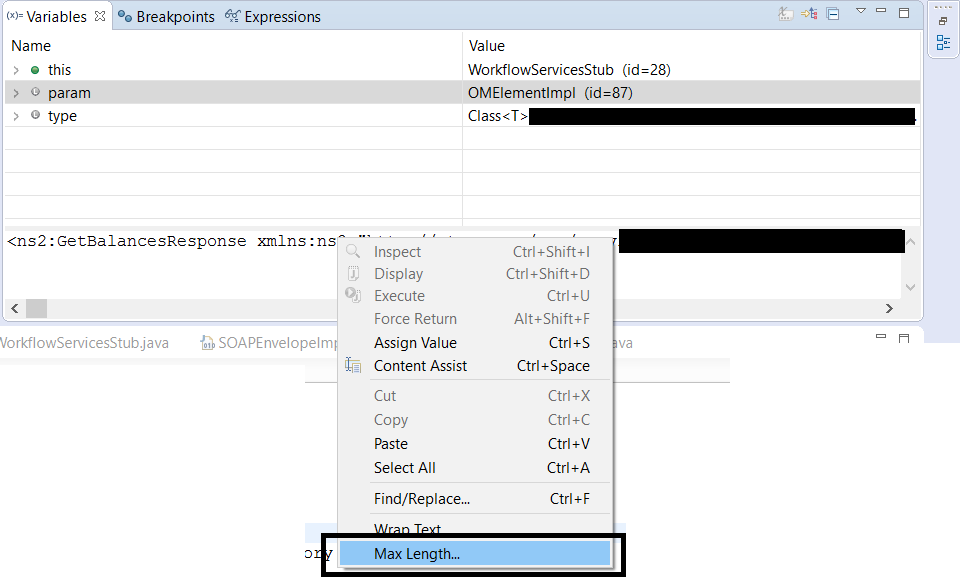
Thats it guys, enjoy coding.
Tags:debug, eclipse, java, max length, variables
Posted in Eclipse, java, Uncategorized | Leave a Comment »
January 4, 2017
If any JUnit test cases are configured to execute along Maven build, Eclipse won’t automatically debug them. That means even if you mark breakpoints it won’t stop the execution there. We can enable such debugging with a simple goals parameter :
-DforkMode=never test


If you are using Maven3 Eclipse plugin, go to Debug configurations, Add a new Maven Build configuration, and add the above goal in the Goals input box. By default, Maven runs tests in a separate process. When you set forkMode
option to never
, maven plugin will not fork the test process. Hence, while connected to the build process you can debug it. The executions will be stalled at the breakpoints as well.
Happy Coding Guys.
Posted in Uncategorized | Leave a Comment »
July 11, 2012
The Ten Commandments of Egoless Programming:
-
Understand and accept that you will make mistakes. The point is to find them early, before they make it into production. Fortunately, except for the few of us developing rocket guidance software at JPL, mistakes are rarely fatal in our industry. We can, and should, learn, laugh, and move on.
-
You are not your code. Remember that the entire point of a review is to find problems, and problems will be found. Don’t take it personally when one is uncovered.
-
No matter how much “karate” you know, someone else will always know more. Such an individual can teach you some new moves if you ask. Seek and accept input from others, especially when you think it’s not needed.
-
Don’t rewrite code without consultation. There’s a fine line between “fixing code” and “rewriting code.” Know the difference, and pursue stylistic changes within the framework of a code review, not as a lone enforcer.
-
Treat people who know less than you with respect, deference, and patience. Non-technical people who deal with developers on a regular basis almost universally hold the opinion that we are prima donnas at best and crybabies at worst. Don’t reinforce this stereotype with anger and impatience.
-
The only constant in the world is change. Be open to it and accept it with a smile. Look at each change to your requirements, platform, or tool as a new challenge, rather than some serious inconvenience to be fought.
-
The only true authority stems from knowledge, not from position. Knowledge engenders authority, and authority engenders respect – so if you want respect in an egoless environment, cultivate knowledge.
-
Fight for what you believe, but gracefully accept defeat. Understand that sometimes your ideas will be overruled. Even if you are right, don’t take revenge or say “I told you so.” Never make your dearly departed idea a martyr or rallying cry.
-
Don’t be “the coder in the corner.” Don’t be the person in the dark office emerging only for soda. The coder in the corner is out of sight, out of touch, and out of control. This person has no voice in an open, collaborative environment. Get involved in conversations, and be a participant in your office community.
-
Critique code instead of people – be kind to the coder, not to the code. As much as possible, make all of your comments positive and oriented to improving the code. Relate comments to local standards, program specs, increased performance, etc.
Courtsey : http://blog.stephenwyattbush.com/2012/04/07/dad-and-the-ten-commandments-of-egoless-programming
Posted in Uncategorized | Leave a Comment »
April 26, 2012
I got one requirement where I will draw one custom component and it should glow when we mouse hover it and remove glow when move the mouse out. This is a simple thing, though I am putting this so that you can save time. 🙂
First we need to write a function which will be triggered on both mouse hover and mouse out of the component. Obviously it will accept one MouseEvent. Inside that we need to create one GlowFilter and add this filter to the component. Set the properties for the flow filter such as color, alpha and quality. On mouse out am removing all the filters. This will work for me since I do not have any other filters for the component. If you have other filters, then iterate through the filters and remove only the glow filter. The function will look like below :
private function highLightCircle(event:MouseEvent):void{
var glowFilter: GlowFilter = new GlowFilter();
var circ:Object = event.currentTarget;
if(circ.filters.length == 0){
glowFilter.color = 0xFFFFFF;
glowFilter.alpha = 1;
glowFilter.blurX = 10;
glowFilter.blurY = 10;
glowFilter.quality = BitmapFilterQuality.HIGH;
circ.filters = [glowFilter];
}else{
circ.filters = null;
}
}
Now we need to call this on mouse over and mouse out. Just add the event listeners to the component object on which you need to apply the filter like below.
circle.addEventListener(MouseEvent.ROLL_OVER, highLightCircle);
circle.addEventListener(MouseEvent.ROLL_OUT, highLightCircle);
Thats all guys. Flex your life. Happy Coding. 🙂
Tags:actionscript, Adobe Flex, apache flex, component, flex, glow filter, glow filter to component in flex
Posted in Uncategorized | Leave a Comment »
August 23, 2011
One of the best articles I had seen so far on decorator pattern
Introduction This series of articles will help you to build a good understanding of design patterns using different examples from real life and some from well-known frameworks or APIs. There are many articles around the web that discuss design patterns but they sometimes lack appropriate examples to quote. So, either their purpose stays unclear or we cannot memorize the patterns longer and sooner they slip out of mind. More importantly, we unders … Read More
via Zeeshan Bilal’s Blog
Tags:decorator-pattern, decorator-pattern-2, design, design-pattern, design-pattern-2, design-patterns-by-examples, design-patterns-by-examples-2, pattern
Posted in Uncategorized | Leave a Comment »